You can use the "Macros" menu items or tool buttons to record and play macros. If you only need a temporary macro you can record a quick macro.
If you want to create a more advanced macro - you can do so by manually "edit" the macro. The macro editor support syntax highlighting and indention.
The macro editor
Macros are saved as values in a list. The first number on a line is the command. The rest are values separated by a tab. The value can be a number or a string.
The macro editor will display the command numbers as command names, automatically indent lines and use different highlight colors to make the list more readable.
NOTE! Your own indentions are not saved. Indention is done automatically since no source code is saved. Only a list of numbers and values.
A sample macro could look like this:
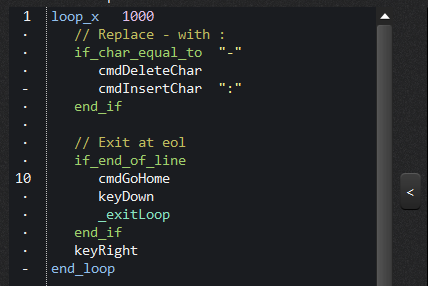
The macro above will check all characters on the line and replace "-" characters with ":". After it reaches the end of line, the cursor is moved to the beginning of the next line. This is where the macro ends.
(The indention you see above is automatically added when you open the macro.)
Commands, loops, variables and if..else statements
Loops
There is only one type of loop. The number added after loop_x can be any positive integer value. If you need to exit the loop before the count is done - you can use the _exitLoop command.
loop_x <tab> number
Start a loop and set how many times you want the macros inside the loop to be executed. You must separate the loop_x command and the number with a tab.
end_loop
This is where the loop ends. Only macros up to this point will be executed, unless you have exited the loop.
_exitLoop
Use this command to exit the loop. The execution will jump to after the "end_loop" command.
Empty lines and comments
You are allowed to use empty lines and line comments // in the macro. Those lines are simply ignored when playing the macro.
You can only add a comment to an empty line. Comments are not allowed after a command.
if .. else .. endif
To create an advanced macro you will probably need to be able to test certain conditions. E.g. if the find command actually found something or if the character under the text cursor is a number.
if_char_equal_to
if_char_greater_than
if_char_lesser_than
Compare the character under the text cursor with the given character.
Ex.
if_char_greater_than <tab> "z"
// do something...
end_if
if_char_is_number
Test if the character at the text cursor is a number.
if_char_is_alpha
Test if the character at the text cursor is a letter.
if_char_is_lowercase
Test if the character at the text cursor is lower cased.
if_char_is_uppercase
Test if the character at the text cursor is upper cased.
if_end_of_line
Test if we have reached the end of the line.
if_end_of_file
Test if we have reached the end of file.
Ex.
if_end_of_file
// We have reached the end. Exit the macro.
_exitMacro
end_if
if_found_string
Use after a find command e.g. cmdFindString "dummy" to see if we found something.
if_selection
Test if we have a selection.
if_var_equal_to
if_var_greater_than
if_var_lesser_than
It is possible to use a variable in a macro. The variable type is integer.
These if types can be used to test the value of the variable.
Ex.
var_int 1
loop_x 10000
if_var_lesser_than 10
varIntToClipboard
cmdPasteFromClipboard
else_do
// do something
end_if
varIncrement 1
keyDown
if_end_of_file
_exitLoop
end_if
end_loop
else_do
If the if test fails, do something else.
end_if
This ends the if structure.
Options (or modes)
There are a few edit modes you can switch between inside a macro.
opt_SelectionModeOn
opt_SelectionModeOff
opt_ColumnModeOn
opt_ColumnModeOff
opt_InsertMode
opt_OverwriteMode
The options are pretty self explaining and enable you to switch between overwrite mode, column mode and selection mode.
Selection mode makes it simple to make a selection. Turn it on and move the text cursor. All text between the first position and the last is now selected.
Commands
Below is a list of all commands.
cmdBackspace
Backspace. Delete the character to the left of the text cursor.
cmdCapitalize
Capitalize selected text.
cmdCaretMoveX <tab> nr
Move the text cursor left or right nr of steps.
cmdCaretMoveY <tab> nr
Move the text cursor up or down nr of steps.
cmdClearClipboard
Clear the contents of the clipboard.
cmdClearSelection
De-select the text.
cmdColumnDelete
Delete the entire selected column.
cmdColumnInsert <tab> "string"
Insert a string to every selected column.
cmdCompressLines
Compress selected lines.
cmdCopyAndAppendToClipboard
Append the selected text to the clipboard.
cmdCopyToClipboard
Copy selected text to clipboard.
cmdCutAndAppendToClipboard
Cut the selected text and append it to the clipboard.
cmdCutToClipboard
Cut selected text to clipboard.
cmdDeleteBlankLines
Delete empty or blank lines. If no selection is made this affect the entire document.
cmdDeleteChar
Delete the character at the text cursor.
cmdDeleteDuplicateLines
Remove duplicate lines in a selection, or the entire file if nothing is selected.
cmdDeleteLine
Remove the current line.
cmdDeleteSelection
Delete the selected text.
cmdDuplicateLine
Duplicate the current line.
cmdDuplicateLineNTimes <tab> nr
Duplicate the current line nr number of times.
cmdFindAll <tab> nr "string"
NOTE! This command is intended to be used internally when recording a find all.
Find all instances of the search string. The nr value set the search options. To set the options add the values below:
1 - case sensitive
2 - match whole words
4 - regular expression
8 - skip tags
16 - start from cursor
32 - search inside a selection
Ex.
Find all instances of "dummy", match whole words only, case sensitive and start from cursor pos:
cmdFindAll 19 "dummy"
(16 + 2 + 1 = 19)
cmdFindNext <tab> nr
Find next occurrence downwards. The nr value is used to set the find options. Add values below to calculate a number.
1 - case sensitive
2 - match whole words
4 - regular expression
8 - skip tags
cmdFindPrevious <tab> nr
Find the next occurrence upwards. The nr value is used to set the find options. Add values below to calculate a number.
1 - case sensitive
2 - match whole words
4 - regular expression
8 - skip tags
cmdFindString <tab> nr <tab> "string"
Find the search string. The nr value is used to set the find options. Add values below to calculate a number.
1 - case sensitive
2 - match whole words
4 - regular expression
8 - skip tags
Ex.
cmdFindString 3 "dummy"
3 = 1 + 2 (case sensitive + match whole words).
cmdGoDocEnd
Move the text cursor to the end of the document. This is the same as Ctrl+End.
cmdGoDocHome
Move the text cursor to the beginning of the document. This is the same as Ctrl+Home.
cmdGoEnd
Move the text cursor to the end of line.
cmdGoHome
Move the text cursor to the beginning of the line.
cmdGoLine <tab> nr
Goto the line entered as nr. E.g. cmdGoLine 120.
If "nr" is empty a dialog box is opened where you can input the line number.
cmdGotoNextBookmark
Move the text cursor to the next bookmark.
cmdGotoNumberedBookmark <tab> nr
Goto a numbered bookmark. "nr" must be a number from 0 to 9.
cmdGotoPrevBookmark
Move the text cursor to the next bookmark upwards.
cmdGoWordNext
Move the text cursor to the next word (Ctrl+Right).
cmdGoWordPrevious
Move the text cursor to the previous word (Ctrl+Left).
cmdInsertChar <tab> "<char>"
Insert a given character at the text cursor position.
Ex.
cmdInsertChar "&"
cmdInsertIndentedText <tab> "string"
Insert text at the text cursor position and preserve indention if string contain newlines.
(This command should probably only be used internally when recording a macro)
cmdInsertString <tab> "string"
Insert text at the text cursor.
cmdInsertTab
Insert a tab at the text cursor position.
cmdInsertTag <tab> nr <tab> "string"
NOTE! This command is intended to be used internally when recording an insertion of HTML tags using auto completion.
cmdInsertTime
Insert the current time at the text cursor.
cmdInvertCase
Invert case of the selected text or the character at the text cursor.
cmdJoinLines
Join selected lines into one line, or join the current and the next if no selection is made.
cmdLowerCase
Lower case of the selected text or the character at the text cursor.
cmdMoveLinesDown
Move selected lines or the current line downwards one step.
cmdMoveLinesUp
Move selected lines or the current line upwards one step.
cmdNewLine
Add a new line.
cmdOverwriteChar <tab> "<char>"
Overwrite the character at the text cursor with given character.
Ex.
cmdOverwriteChar "?"
cmdPasteFromClipboard
Paste text from clipboard at the text cursor position.
cmdReplaceAll <tab> nr "<find string>"+#160+"<replace string>"
NOTE! This command is intended to be used internally when recording a replace all.
Replace all instances of the search string. The nr value set the search options. To set the options add the values below:
1 - case sensitive
2 - match whole words
4 - regular expression
8 - skip tags
16 - start from cursor
32 - search inside a selection
Ex.
Match all instances of "dummy", match whole words only, case sensitive and start from cursor pos:
cmdReplaceAll 19 "dummy<#160>jummy"
(16 + 2 + 1 = 19)
cmdReplaceWith <tab> "string"
Use with cmdFindString to replace a text string.
Ex.
cmdFindString 3 "dummy"
if_found_string
cmdReplaceWith "Lorem ipsum"
end_if
cmdSelectAll
Select all text (Ctrl+A).
cmdSelectDn
Select down (Shift+Down).
cmdSelectEnd
Select to the end of file (Shift+Ctrl+End).
cmdSelectHome
Select to the beginning of file (Shift+Ctrl+Home).
cmdSelectLeft
Select left (Shift+Left).
cmdSelectLineEnd
Select to the end of line (Shift+End).
cmdSelectLineHome
Select to the beginning of line (Shift+Home).
cmdSelectPageDn
Select page down (Shift+PageDown).
cmdSelectPageUp
Select page up (Shift+PageUp).
cmdSelectParagraph
Select current paragraph.
cmdSelectRight
Select right (Shift+Right).
cmdSelectScreenLineEnd
If the text line is wrapped, select to the end of the row.
cmdSelectScreenLineHome
If the text line is wrapped, select to the beginning of the row.
cmdSelectSentence
Select the current sentence.
cmdSelectSentenceEnd
Select to the end of the current sentence.
cmdSelectSentenceHome
Select to the beginning of the current sentence.
cmdSelectUp
Select up (Shift+Up).
cmdSelectWordLeft
Select word left (Shift+Ctrl+Left).
cmdSelectWordRight
Select word right (Shift+Ctrl+Right).
cmdSmartPasteFromClipboard
Paste from clipboard and preserve the current indention (Shift+Ctrl+V).
cmdSortAsc
Sort the selected text, or entire document if nothing is selected, in ascending order.
cmdSortDesc
Sort the selected text, or entire document if nothing is selected, in descending order.
cmdSortNumericAsc
Sort the selected text, or entire document if nothing is selected, based on found numbers on the line in ascending order.
cmdSortNumericDesc
Sort the selected text, or entire document if nothing is selected, based on found numbers on the line in descending order.
cmdSplitLines
Split lines at the right margin (or 80). If nothing is selected only the current line is split.
cmdStringToClipboard <tab> "string"
Add the string to clipboard.
cmdUpperCase
Upper case the selected text of the character at the text cursor.
keyCtrlEnd,
keyCtrlHome,
keyDown,
keyEnd,
keyHome,
keyLeft,
keyPageDown,
keyPageUp,
keyRight,
keyUp
Move the text cursor. E.g. keyLeft will move the text cursor one step to the left.
var_int <tab> nr
Give the variable an integer value.
varDecrement <tab> nr
Increment the variable value by nr. If no value is given the variable is incremented by 1.
varIncrement
Decrement the variable value by nr. If no value is given the variable is decremented by 1.
varIntToClipboard
Copy the variable to the clipboard as a text string. E.g. 100 is copied to the clipboard as "100".
Display a dialog window
showColorDlg
Display the color dialog box, select a color and insert at the text cursor.
showInsertAscNumbersInColumns
This dialog window is used in column mode to add ascending numbers at the beginning of the selected lines.
showHtmlButtonDlg,
showHtmlCSS,
showHtmlFontDlg,
showHtmlFormDlg,
showHtmlFrameDlg,
showHtmlInsertDlg,
showHtmlListDlg,
showHtmlMetaDlg,
showHtmlAnchorDlg,
showHtmlImageDlg,
showHtmlTableDlg,
showHtmlTextAreaDlg
HTML help dialog windows to create HTML tags.
|